From the Journal
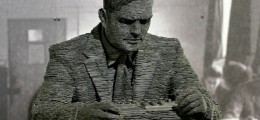
Thank You Alan Turing
June 23rd will mark the 100th anniversary of the life of famous English mathematician Alan Turing.
Within the Google Code Labs lies the Social Graph API that can be used to display all your connections on the net. Instead of having to go find all the links to the different social networking sites you belong to you can let this API do the heavy lifting for you. Let’s see how we can use this API and some JQuery to create a list of “Places you can find me”, as well as, pull in our latest tweet from Twitter.
First we’ll start with a basic piece of HTML markup:
<html dir="ltr" lang="en"> <head> <title>Makin' It Happen with jQuery & Google's Social Graph API</title> <style type="text/css"> body {font-family: Georgia, Serif;font-size:12px;} h2 {font-family: "Helvetica Neue", Arial, Helvetica, Sans-Serif;font-size:20px;} ul {list-style:none;margin:0;padding:0;width:250px;} li {margin:0;padding:0;float:left;width:250px;} li img, li a {float:left;margin:6px 3px;display:block;} </style> </head> <body> <h2>Most Recent Tweet</h2> <div id="tweets"></div> <div id="social"></div> </body> </html>
Pretty standard setup here. We’ve got two empty DIVs for our tweet and our social links. Next we’ll need to add the JQuery library from the Google Libraries API to the
of the file. In order to do this you’ll need to have an API Key provided from Google.
<script type="text/javascript" src="http://www.google.com/jsapi?key={YOUR KEY HERE}"></script> <script language="Javascript" type="text/javascript"> //<![CDATA[ google.load("jquery", "1"); //]]> </script>
Using this method is nice, because the library is being loaded off of your website, and allows for some easier maintenance in my opinion. Let’s go ahead and add the code to pull our latest tweet from Twitter. This will go directly after the scripts for the Google Libraries API.
<script type="text/javascript> $(document).ready(function() { $.getJSON("http://twitter.com/status/user_timeline/{TWITTER USERNAME HERE}.json?count=1&callback=?", function(data) { $("#tweets").html(data[0].text); }); }); </script>
Since the Twitter API uses JSON for it’s responses, we’ll want to use JQuery’s getJSON function to deal with this information. What we’ve basically told the API is: “We’d like the last tweet from this Twitter user, and we’d like the information displayed within the DIV with ID ‘tweets’ please”. Naturally, we’ll wrap it all up in a document.ready function so that this code will wait until the page is ‘ready’ before executing. One line of code and we’re done. We can always go back and style the text appropriately, but for now this gets us where we want to be.
Next we’ll add the line that will make the call to the Social Graph API. This will be wrapped within the same document.ready function as our Twitter API call.
<script type="text/javascript> $(document).ready(function() { var cbscript = document.createElement("script"); cbscript.src = 'http://socialgraph.apis.google.com/lookup?q=pixelhavenllc.com&fme=1&edi=1&edo=1&callback=ShowLinks'; cbscript.type = 'text/javascript'; document.body.appendChild(cbscript); $.getJSON("http://twitter.com/status/user_timeline/{TWITTER USERNAME HERE}.json?count=1&callback=?", function(data) { $("#tweets").html(data[0].text); }); }); </script>
Basically what this is doing it appending a <script> to the <body> of a page that makes the call to the API. I’ve used the lookup method for this example, because it is really the simpler method to use for low-level access to the API.
Red Hot Chili Peppers - Under the Bridge
Blind Melon - Change
Tracy Chapman - Give Me One Reason
Third Eye Blind - How's It Going To Be
Sugar Ray - Every Morning